Home » Resources » How-to Guides » Marketo Installation Hub » Marketo Forms Installation
Marketo Forms
Our Marketo Forms integration allows you to add validation and address capture to your forms, through a callback function in the embed form code.
Step 1: Modify the embed code to use the built-in callback function
If you haven’t already, get your Marketo forms embed code from the Form Actions drop down on their site.
The default embed form code looks something like this:
To implement the callback function, this code should be changed like so:
jQuery is required for our integration to work. If you aren’t sure how to add it to your page, the following will work:
Step 2: Generate your configuration code using the form below
Simply fill out the form and click generate to get your custom code..
An API key is required to use any of the validation or PredictiveAddress services. You can generate one from the Dashboard, under the Validation tab.
Step 3: Paste code
Having generated your code above, you can now copy and paste this into your form embed code that we prepared in step 1.
You should insert the code into your callback function, replacing the //insert Data8 code here
line with the generated code.
Please note that if you are using the PredictiveAddress service you will need to replace the field id’s (address1
, address2
etc) with your address field id’s. For help on how to do this, see the ‘Advanced Configuration’ section below.
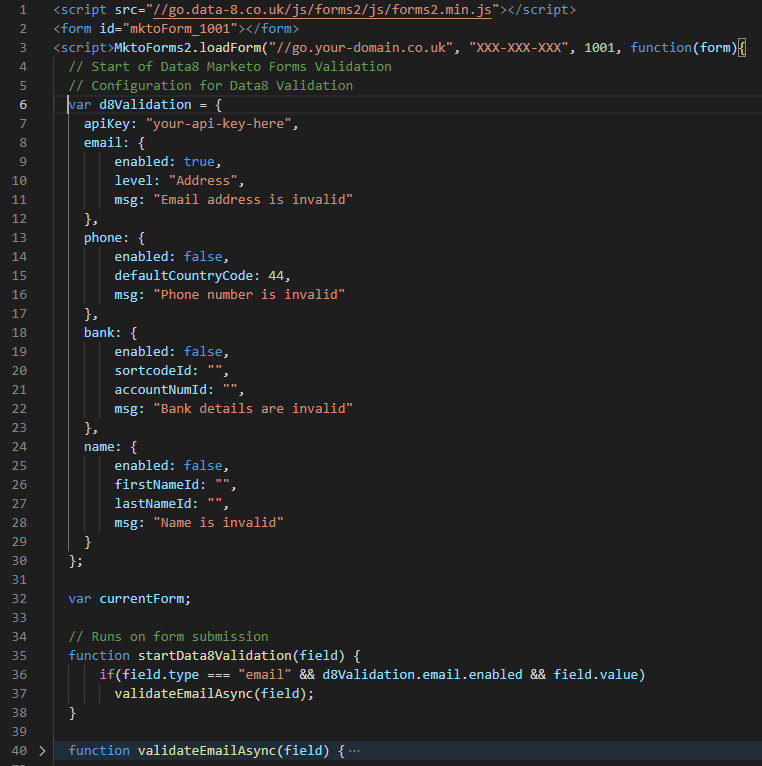