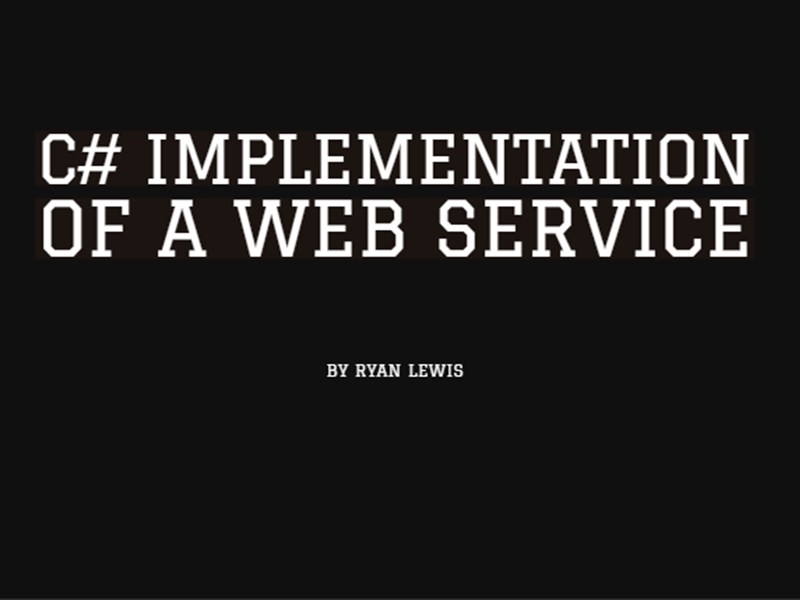
Every day is a learning day in data and web development. The following blog is from Ryan who recently joined the data8 team. His enthusiasm for the role comes through and willingness to learn as well!
“…The aim of this post is to show my journey step-by-step, from learning the basics of web development, right through to creating a working web application with an email validation check.
I began working at Data-8 at the start of January and coming from a Game Development background I had no real experience developing in MVC or developing web applications. I quickly felt like I would be out of my depth, but within a week of being at the company, I was introduced to one of our webservices, ‘Email Validation’, and was tasked with implementing it into my first web form application.
The web form application would be for a library book request system, where users would leave a name and email address as they add themselves to a waiting list for the book they specify. In a real system, the validity of the email address would be important to ensure that the user could be contacted when the book is ready.
*Looking back, this was a very enjoyable first project and I picked it up easily which gave me a huge boost in confidence. I’d also like to point out that Telerik Fiddler was a very useful tool whilst trying to implement the webservice, as it allowed me to get a better understanding of what was happening behind the scenes. *
The first step towards creating a web application to implement a web service through C# and MVC, was to create an ASP.NET Web Application project in Visual Studio.
Creating A Controller for The Home Page Including Actions
Next, I needed to create a controller to link a model (like a class for data) to a view (user interface) and process the inputs from the view.
To create a controller, I right clicked on the controllers’ folder in the solution explorer > add controller (I named it Home Controller)
Creating A Service Reference for the Email Validation Web Service
Next, I discovered that I needed to create a service reference for the web service that I wanted to implement. This would allow my code to access the API and use the functionality of the service (at the time it was just something I had to do to get it to work).
In solution explorer, I right clicked on References > Add Service Reference > and in the Address box added the link to the web service (e.g. http://webservices.data-8.co.uk/emailvalidation.asmx)
I clicked Go > Selected the drop-down icon next to the service in the services box > Selected the option with -Soap at the end > and saw a list of available operations reassuring me that I’d done it correctly.
I named the service reference something appropriate (‘EmailValidationWS’) and clicked ‘OK’.
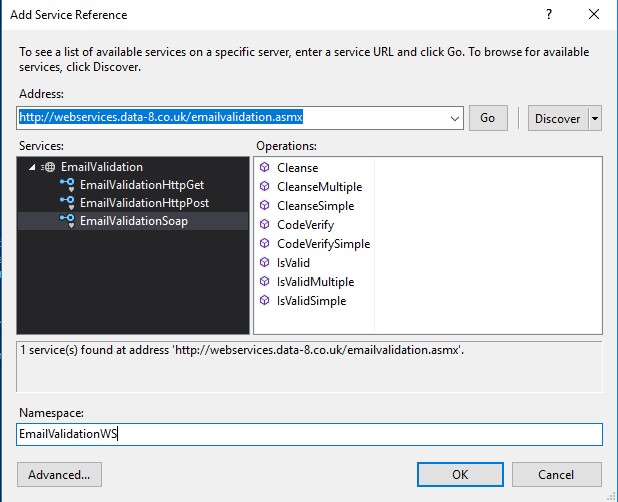
The next step was to add some code to my controller to make it come to life. I began by creating ‘ActionResult’ functions for each view, which could return the model data to a view for the user to see. I also created an overloaded function for my main view (‘RequestABook’). The overloaded function took a model (that I would later create) as a parameter, and then used the data from the model to validate the email address.
I created a reference to the email web service soap (Simple Object Access Protocol), and then used that reference to call a function on the web services API. In this instance I called the ‘IsValid’ function, which took a username, password, email address to validate, validation level enum and any extra options. The function returned a bunch of data, but the bit I was interested in was the Result object, which would inform me if the email address was Valid or Invalid.
I did a quick check on the validity of the email address, and if it was invalid then I outputted an error message through the ‘ModelState’. Otherwise, it would take the user to a view called ‘ThankYou’.