Marketing Manager at Data8
JavaScript Implementation of a Web Service

The latest blog from Ryan and his learning. The aim of this post is to show my journey step-by-step, taking my prior creation of a C# MVC web application with an email validation check, and using the learned knowledge to create a front-end (JavaScript and HTML) web application with an email validation check. Again, front-end development was something that I had very little experience with before this project.
This project was my second task at Data-8, and I completed it within my second week of starting at the company.
My first step to implementing a web service through the front-end was to create an ASP.NET Web Application project in Visual Studio.
Creating A View with HTML or Razor HTML to Gather Inputs from the User
The only page I would need for front-end implementation would be a view, as this is where both JavaScript and HTML are written.
In solution explorer, I opened the Views folder > Home > Index
I cleared out the existing ‘startup’ HTML and added the following code:
This created a basic form with input fields to gather information about a Book Name, Email Address and Contact Name. These would then be used later on in the validation checks to collect user data to validate. The alert div element is currently hidden (style=”display:none;”) but can be shown later on in code by calling .show(); I used this to output the result of validation checks in a nice way.
Creating a Small JavaScript Function to Test the Submit Button Functionality
My next step was to create a basic function that would be called when the user clicked the submit button. I only wanted to test functionality, so I implemented an alert to output ‘Clicked’.

I ran the program and saw the three fields and a button. I clicked the button and got the expected result of an alert displaying ‘Clicked’
Adding ‘OnBlur’ To Produce Alerts as Soon as the User Clicks Off A Text Box
The next thing I wanted to implement was to perform validation checks on the entered data as soon as the user clicked off a field. This instant feedback would be useful as it catches errors in entered data before the user submits the form.
After researching how to trigger an event when the user clicks off a box, I came across the ‘onblur’ event. This was implemented as an attribute to a HTML element and pointed to a function that should be called when the user clicks off that HTML element.
I created a function called ‘OnFocusChanged’ which took two parameters, an ID and a value of the entered data. This would populate the hidden alert message every time the user clicks off a field in the form without entering any data. It generates a message based off the ID of the element that was active, and outputs it to the hidden alert box. Notice the ‘.show()’ at the end of the jQuery line that reveals the html element to the user.

I also added the ‘onblur’ code into the HTML elements and passed in the ‘OnFocusChanged()’ function to be called when the user clicks off the field. I gave the function parameters of a string to identify the HTML element and the data that was entered.

I then built the program which displayed the three input fields and a submit button. Clicking into a field and then clicking off it should generate an error message for the respective field.

Make an Ajax Request to Check the Validity of the Data on Submit Clicked
So far, I had created a program that ensures that the user has entered data before clicking the ‘Submit’ button. However, it doesn’t use any web services to check the validity of the entered data, only that some entered data exists.
The next step was to implement a simple validation check in the ‘OnSubmitClicked’ function to check that the required data is not empty, before I pass it into a web service call. I used jQuery to access each required field’s value, performed a check if the value was empty, and if so, outputted a message to inform the user that they had to enter data in that field. It also returns, which stops the function from performing any attempt at validation checks.
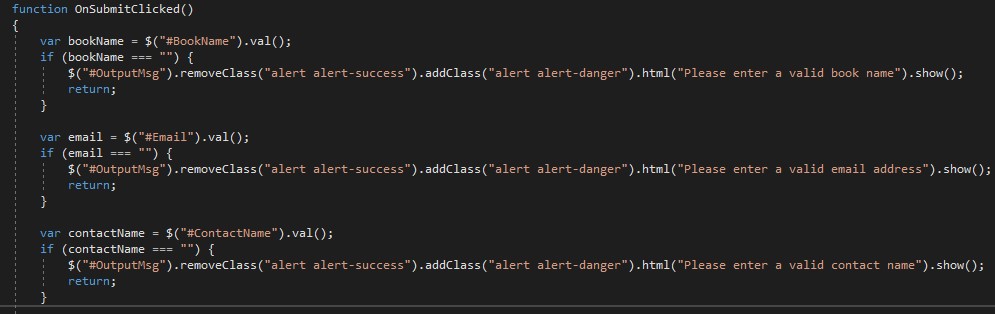
Once the data had passed the null/empty checks, it needed to be passed into a web service call to validate the entered data. To call the webservice, I created an Ajax request which passed data in a formatted structure, to an API and then received formatted data in return as a result of the check.
For this project, I wanted to use the Email Validation web service by Data-8 to check the validity of the entered email address.
This ajax request would generate a message upon a successful ajax request. The message is generated from a switch function which checks the result of the validation check.
When attempting to implement the web service, I found I was getting errors from the way in which I was passing data into the call. I researched further into the webservice and found several pages of documentation which helped me understand the requirements.

Finding Out the Data Structure Requirements of the Input and Output of a Web Service
To find out what data would be returned, or the structure of the data passed into or returned from the web service call, I had to access the web services documentation for the email validation web service and digest the XML code to find the structure of the data.
For example, with email validation (http://webservices.data-8.co.uk/emailvalidation.asmx):

On the ‘Email Validation’ service documentation page, I saw a list of all the email validation checks that I could call. I chose ‘IsValid’ which was the function I would be calling in my project.
I was then presented with several blocks of XML code. I found the data structure for data being passed in to the ajax request in the blue circled area under the name of the function being called.
The data structure for the data returned from the ajax request was in the second screenshot of XML code, highlighted in red and was inside an object called the function name followed by Result (e.g. ‘IsValidResult’).

In the ‘Level’ and ‘Result’ variables, multiple possibilities for data can be seen representing an Enum. All possible options are listed, but only one needed to be used at a time, and it needed to be entered exactly as shown, including capitalisation and as a string literal.
Back to the Ajax Request and Web Service Call
Now that I had an understanding of the structure of the data I was entering and getting back, I could see where I was going wrong. I formatted the data before passing it as a ‘JSON String’ (with ‘JSON.Stringify()’) into the function. The entered data needed a name as a string, and a value.

When I now built the program, entered a value in all fields, and clicked ‘Check’, the alert banner produced a result of the validity of the entered email address.

My program had a basic input validation for any fields marked as required, and also a validation check on the entered email to check it was valid. This was another extremely useful project that taught me a lot about front-end development including jQuery, HTML coding, and Ajax Requests, and how Data-8’s web services worked and how to know what data is required for the validation check.
For more help, just check out our section "How to" on the website. Thank you for reading and if you have any questions, please don't hesitate to ask. Ryan.
Find out more about how Data8 can help you